is on your own risk !!
For any new CBDBv2 Evo orders/requests please feel free to use as usual:
tech at esp8266-projects.com.
If you want for your own experiments to order CBD v2 EVO bare PCBs only, you can also do it directly at Dirty PCBs, our preferred PCB House:
http://dirtypcbs.com/view.php?share=5876&accesskey=3d0fd70d53d6dc20c0bf0255f67cde65
——————————————————————————————————————
As promised, today we will continue with our Live Battery Monitor System.
Subjects of the day:
- WebServer interface for direct access
- Live Data upload over the Internet for wordwide access on Thingspeak.com.
What we will need:
- CBDB Board ( or any other ESP8266 Board you may like but who has voltage/current measurement capabilities)
- INA214 – Current shunt monitor
- USB adapter (take a look on Part 1 for details about the USB Adapter )
- Li-Ion Battery Module
- 20 Ω / 5W resistor (or 2 x 10 Ω /5 W in series – even better )
- Connection wires – various colors
For schematics, theory of operation and technical related things please see previous article: Battery Live Monitor System
Live monitoring data, with 10 and 20ohm resistor Load:
For programming CBDBv2 Board and uploading the drivers and the software we will continue to use the LuaUploader as before. 1. Define used GPIO pins and variables:
ADC_SRC = 5 -- GPIO14 - select Voltage Divider / Current Inputsda=2 -- GPIO4 - SDA scl=1 -- GPIO5 - SCL gpio.mode(ADC_SRC,gpio.OUTPUT, gpio.PULLUP) gpio.write(ADC_SRC,1) -- Voltage Measurement - Voltage Divider Source selected gpio.write(ADC_SRC,0) -- Current Measurement - Current Shunt Monitor output selectedvoltdiv= 0.00412 -- Voltage reading calibration dival = 0.00096 -- ADC volt/div value - CALIBRATE !! resdiv = 4.31447 -- Voltage Divider Ratio - CALIBRATE!! divalI = 0.9425 -- Current volt/div ratio - CALIBRATE!! cpct = 0 -- Calculated Delivered Energy adcI = 0 -- ADC readings - Curent adcV = 0 -- ADC readings - Voltage pwr = 0 -- Calculate Power t=0 -- time
2. READ ADC Process function and calculations
function readUI() gpio.write(ADC_SRC,1) advr=adc.read(0) adcV=advr*dival*resdiv print("\nVoltage : " ..string.format("%g",adcV).." V") tmr.delay(10000) gpio.write(ADC_SRC,0) adcr=adc.read(0) adcI=adcr*divalI print("Current : " ..string.format("%g",adcI).." mA") --instantaneous Power consumption calculation pwr = adcI*adcV print("Power : " ..string.format("%g",pwr).." mW") -- total delivered Energy calculation if (adcV > 1) then cpct=cpct+pwr*0.002778/1000 t = t +1 print("Energy : " ..string.format("%g",cpct).." W") else tmr.stop(0) dtime = t/360 -- calculate discharge time print("Discharge Time: %.2fh ",dtime) end end
srv=net.createServer(net.TCP) srv:listen(80, function(conn) conn:on("receive",function(conn,payload) --print(payload) conn:send("HTTP/1.1 200 OK\n\n") conn:send("<META HTTP-EQUIV=\"REFRESH\" CONTENT=\"5\">") conn:send("<html><title>Battery Live Monitor System - ESP8266</title><body>") conn:send("<h1>Battery Live Monitor System - ESP8266</h1><BR>") conn:send("Voltage :<B><font color=red size=4>" ..string.format("%g",adcV).." V</font></b><br>") conn:send("Current :<B><font color=blue size=4>" ..string.format("%g",adcI).." mA</font></b><br>") conn:send("Power :<B><font color=red size=4>" ..string.format("%g",pwr).." mW</font></b><br>") conn:send("Energy :<B><font color=green size=4>" ..string.format("%g",cpct).." Wh</font></b><br>") conn:send("<BR><BR><BR>Node.HEAP : <b>" .. node.heap() .. "</b><BR>") conn:send("IP ADDR :<b>".. wifi.sta.getip() .. "</b><BR>") conn:send("TMR.NOW :<b>" .. tmr.now() .. "</b><BR<BR><BR>") conn:send("</html></body>") conn:on("sent",function(conn) conn:close() end) conn = nil end) end)
function sendDataTh() print("Sending data to thingspeak.com") conn=net.createConnection(net.TCP, 0) conn:on("receive", function(conn, payload) print(payload) end) conn:connect(80,'184.106.153.149') conn:send("GET /update?key=YOURKEYHERE&field1="..adcV.."&field2="..adcI.."& field3="..pwr.."&field4="..cpct.."HTTP/1.1\r\n") conn:send("Host: api.thingspeak.com\r\n") conn:send("Accept: */*\r\n") conn:send("User-Agent: Mozilla/4.0 (compatible; esp8266 Lua; Windows NT 5.1)\r\n") conn:send("\r\n") conn:on("sent",function(conn) print("Closing connection") conn:close() end) conn:on("disconnection", function(conn) print("Got disconnection...") end) end
5. MAIN programIf you have a new ESP module or is the first time when using it on a new WIFI network, don’t forget to do the WIFI setup:
-- One time ESP Setup -- wifi.setmode(wifi.STATION) wifi.sta.config ( "YOUR_WIFI_SSID" , "PASSWORD" ) print(wifi.sta.getip())
— Read ADC data every 10s, print values on local Web interface and send them to thingspeak.com
tmr.alarm(0, 10000, 1, function() readUI() sendDataTh() end)
dofile("blmsweb.lua") -- Start the Battery Live Monitoring System print(wifi.sta.getip()) --print your BMS WebServer IP address
![]() |
BLMS – First RUN |
If you want the BLMS software to start automatically when your CBDBv2 Evo Board starts or reboots, then you neet to create and add some lines in your ‘init.lua‘ file:
dofile("blmsweb.lua") -- Start the Battery Live Monitoring System
Save the code on ESP as ‘init.lua‘, restart ESP. It should reboot and restart automatically the program.
Open your favorite Web browser and type your new BLMS Web Server IP address. If all ok, it should look like below :
![]() |
BLMS – Web Server interface – 20ohm Load |
Thingspeak data upload
I will not insist on the Thingspeak.com account setup, it’s a pretty simple process:
- Sign Up for a ThingSpeak account
- Create a new Channel by going to your Channels page and clicking Create New Channel
- Update your Channel via URL:
https://api.thingspeak.com/update?api_key=YOUR_CHANNEL_API_KEY&field1=7
- View your Channel feed:
https://api.thingspeak.com/channels/YOUR_CHANNEL_ID/feeds.json
Take a look at this Tutorial for more details for common devices and applications
![]() |
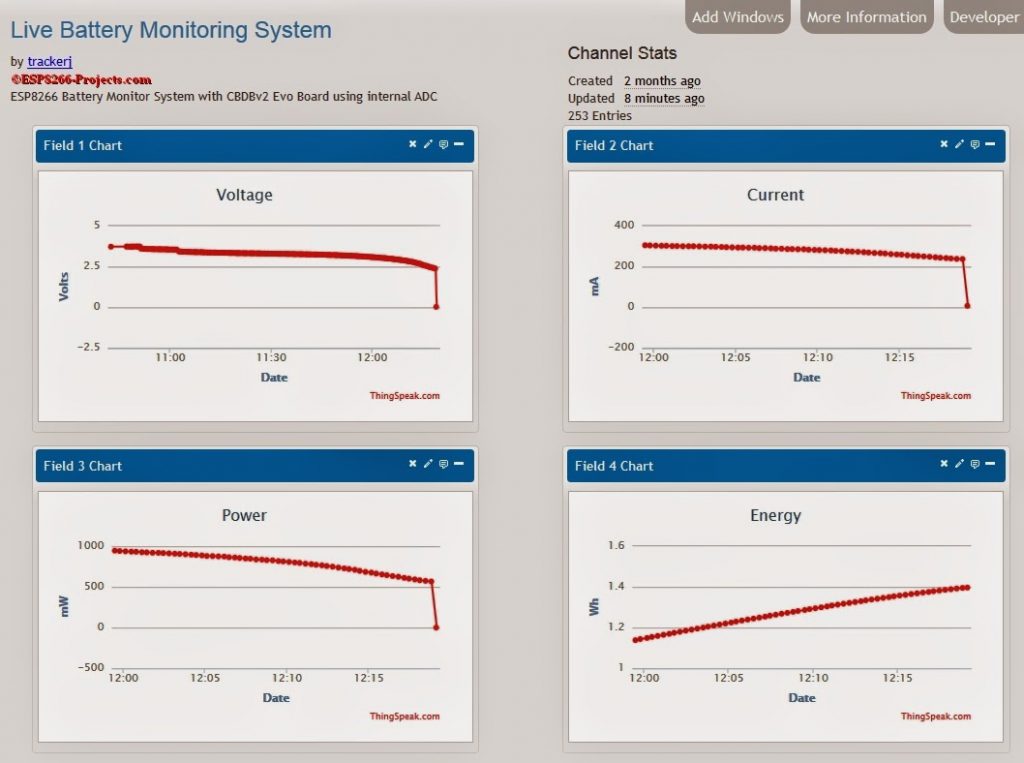